Exemplifying thread context switching – Concurrency – Virtual Threads, Structured Concurrency
215. Exemplifying thread context switching
Remember that a virtual thread is mounted on a platform thread and it is executed by that platform thread until a blocking operation occurs. At that point, the virtual thread is unmounted from the platform thread and it will be rescheduled for execution by the JVM later on after the blocking operation is done. This means that, during its lifetime, a virtual thread can be mounted multiple times on different or the same platform thread.In this problem, let’s write several snippets of code meant to capture and exemplify this behavior.
Example 1
In the first example, let’s consider the following thread factory that we can use to easily switch between the platform and virtual threads:
static class SimpleThreadFactory implements ThreadFactory {
@Override
public Thread newThread(Runnable r) {
return new Thread(r); // classic thread
// return Thread.ofVirtual().unstarted(r); // virtual thread
}
}
Next, we try to execute the following task via 10 platform threads:
public static void doSomething(int index) {
logger.info(() -> index + ” “
+ Thread.currentThread().toString());
try { Thread.sleep(Duration.ofSeconds(3)); }
catch (InterruptedException ex) {}
logger.info(() -> index + ” “
+ Thread.currentThread().toString());
}
Between the two logging lines, we have a blocking operation (sleep()). Next, we rely on newThreadPerTaskExecutor() to submit 10 tasks that should log their details, sleep for 3 seconds, and log again:
try (ExecutorService executor =
Executors.newThreadPerTaskExecutor(
new SimpleThreadFactory())) {
for (int i = 0; i < MAX_THREADS; i++) {
int index = i;
executor.submit(() -> doSomething(index));
}
}
Running this code with platform threads reveals the following side-to-side output:
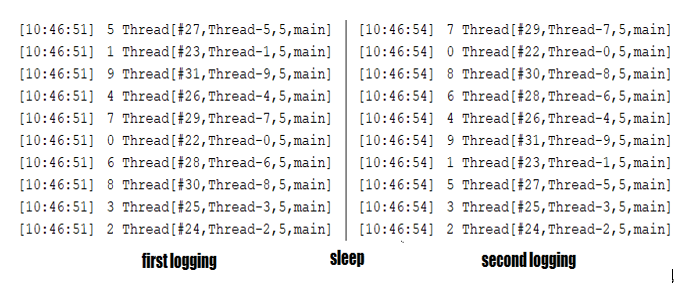
Figure 10.8 – Using platform threads
By carefully inspecting this figure, we notice that there is a fixed association between these numbers. For instance, the task with id 5 is executed by Thread-5, task 3 by Thread-3, and so on. After sleeping (blocking operation), these numbers are unchanged. This means that while the tasks are sleeping the threads are just hanging and waiting there. They have no work to do.Let’s switch from platform threads to virtual threads and let’s run again:
@Override
public Thread newThread(Runnable r) {
// return new Thread(r); // classic thread
return Thread.ofVirtual().unstarted(r); // virtual thread
}
Now, the output is resumed in the following figure:
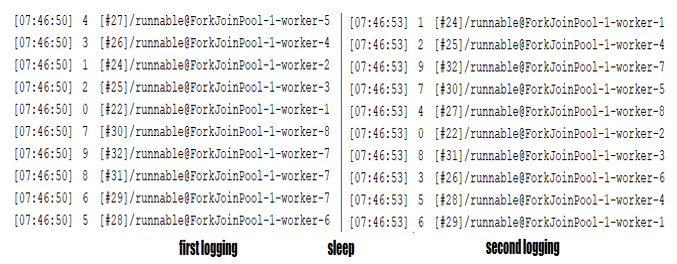
Figure 10.9 – Using virtual threads
This time, we see that things are more dynamic. For instance, the task with id 5 is started by a virtual thread executed by worker-6 but is finished by worker-4. The task with id 3 is started by a virtual thread executed by worker-4 but is finished by worker-6. This means that, while a task is sleeping (blocking operation), the corresponding virtual thread is unmounted and its worker can serve other virtual threads. When the sleeping is over, the JVM schedules the virtual thread for execution and is mounted on another (it could be the same as well) worker. This is also referred to as thread context switching.