Combining StructuredTaskScope and streams 2 – Concurrency – Virtual Threads, Structured Concurrency
And, in the next figure, you can see the event recorded for ending this virtual thread:
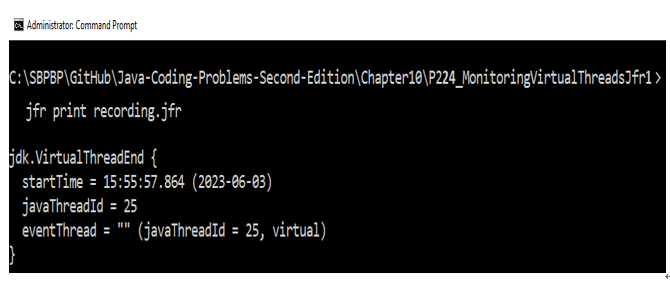
Figure 10.12 – JFR event for ending a virtual thread
Besides the JFR CLI, you can use more powerful tools for consuming the virtual thread events such as JDK Mission Control (https://www.oracle.com/java/technologies/jdk-mission-control.html) and the well-known Advanced Management Console (https://www.oracle.com/java/technologies/advancedmanagementconsole.html).For getting a stack trace for threads that block while pinned we can set the system property jdk.tracePinnedThreads. A complete (verbose) stack trace is available via -Djdk.tracePinnedThreads=full. Or if all you need is a brief/short stack trace then rely on -Djdk.tracePinnedThreads=short.In our example, we can easily get a pinned virtual thread by marking the fetchTester() method as synchronized (remember that a virtual thread cannot be unmounted if it runs code inside a synchronized method/block):
public static synchronized String fetchTester(int id)
throws IOException, InterruptedException {
…
}
In this context, JFR will record a pinned virtual thread as in the following figure:
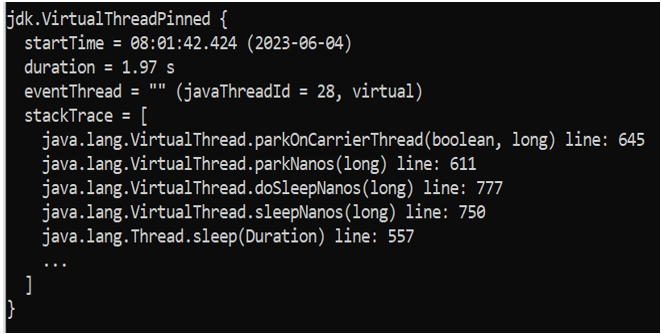
Figure 10.13 – JFR event for a pinned virtual thread
If we run the application with -Djdk.tracePinnedThreads=full then your IDE will print a detailed stack trace that starts as follows:
Thread[#26,ForkJoinPool-1-worker-1,5,CarrierThreads] java.base/java.lang.VirtualThread$VThreadContinuation.onPinned(VirtualThread.java:183)
…
You can see the complete output by executing the bundled code. Of course, you can get a thread dump and analyze it via several other tools. You may prefer any of jstack, Java Mission Control (JMC), jvisualvm, or jcmd. For instance, we can obtain a thread dump in plain text or JSON format via jcmd as follows:
jcmd <PID> Thread.dump_to_file -format=text <file>
jcmd <PID> Thread.dump_to_file -format=json <file>
Next, let’s play with jconsole (JMX) to quickly analyze the performance of virtual threads.
Using Java Management Extensions (JMX)
Until JDK 20 (inclusive), JMX provide support for monitoring only the platform and threads. But, we can still use JMX to observe the performance brought by virtual threads in comparison with platform threads.For instance, we can use JMX to monitor platform threads at each 500 ms via the following snippet of code:
ScheduledExecutorService scheduledExecutor
= Executors.newScheduledThreadPool(1);
scheduledExecutor.scheduleAtFixedRate(() -> {
ThreadMXBean threadBean
= ManagementFactory.getThreadMXBean();
ThreadInfo[] threadInfo
= threadBean.dumpAllThreads(false, false);
logger.info(() -> “Platform threads: ” + threadInfo.length);
}, 500, 500, TimeUnit.MILLISECONDS);
We rely on this code in the following three scenarios.
Running 10000 tasks via cached thread pool executor
Next, let’s add a snippet of code that run 10000 tasks via newCachedThreadPool() and platform threads. We also measure the time elapsed to execute these tasks:
long start = System.currentTimeMillis();
try (ExecutorService executorCached
= Executors.newCachedThreadPool()) {
IntStream.range(0, 10_000).forEach(i -> {
executorCached.submit(() -> {
Thread.sleep(Duration.ofSeconds(1));
logger.info(() -> “Task: ” + i);
return i;
});
});
}
logger.info(() -> “Time (ms): “
+ (System.currentTimeMillis() – start));
On my machine, it took 8147 ms (8 seconds) to run these 10000 tasks using at peak 7729 platform threads. The following screenshot from jconsole (JMX) reveals this information:
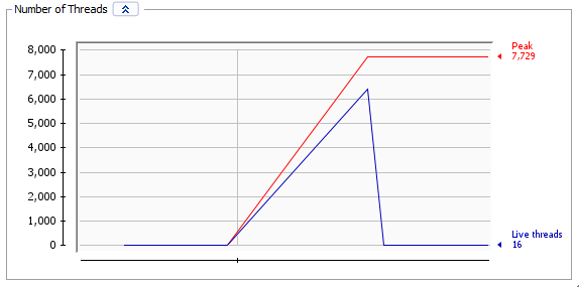
Figure 10.14 – Running 10000 tasks via cached thred pool executor
Next, let’s repeat this test via a fixed thread pool.